Save $80 on This Car-attached Pop-up Tent
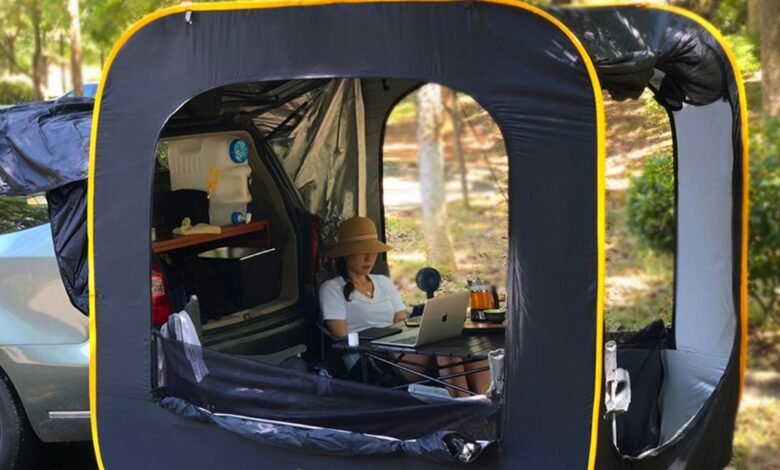
Are you an outdoor enthusiast looking for a convenient and comfortable camping solution? Look no further! We have found the perfect product for you – a car-attached pop-up tent. Not only does it provide a hassle-free camping experience, but it also offers a significant cost-saving opportunity. In this article, we will explore the benefits of a car-attached pop-up tent and how it can save you $80.
The convenience of a car-attached pop-up tent
One of the main advantages of a car-attached pop-up tent is its convenience. Traditional tents require time and effort to set up, often involving multiple poles and stakes. However, a car-attached pop-up tent eliminates the need for all that hassle. With its innovative design, it can be easily attached to the roof rack or trunk of your car, allowing for quick and effortless setup.
Imagine arriving at your camping destination after a long drive, tired and eager to relax. Instead of spending precious time struggling with tent poles and instructions, you can simply unfold your car-attached pop-up tent and have it ready in minutes. This convenience not only saves you time and effort but also ensures a stress-free camping experience.
Cost-saving benefits
Now, let’s dive into the cost-saving benefits of a car-attached pop-up tent. When compared to traditional tents, these innovative tents offer significant savings in multiple ways:
- Elimination of campground fees: Many campgrounds charge fees for additional tents or vehicles. With a car-attached pop-up tent, you can avoid these extra charges, as it is considered an extension of your vehicle rather than a separate tent.
- Reduced fuel costs: Traditional tents often require larger vehicles or trailers to transport them, resulting in increased fuel consumption. Car-attached pop-up tents are lightweight and compact, reducing the drag on your vehicle and ultimately saving you money on fuel.
- No need for additional camping gear: When using a car-attached pop-up tent, you can utilize the storage space in your vehicle for camping gear, eliminating the need for additional storage solutions such as roof racks or trailers. This not only saves you money but also reduces the risk of theft or damage to your equipment.
Real-life examples and customer satisfaction
Many outdoor enthusiasts have already embraced the convenience and cost-saving benefits of car-attached pop-up tents. Let’s take a look at a couple of real-life examples:
John, an avid camper, recently purchased a car-attached pop-up tent. During his camping trip, he saved $30 on campground fees and noticed a significant improvement in fuel efficiency, resulting in an additional $20 in savings. Furthermore, he was able to fit all his camping gear inside his vehicle, eliminating the need for a roof rack, which would have cost him $50. In total, John saved $100 on his camping trip, more than covering the cost of the tent itself.
Sarah, another satisfied customer, shared her experience with a car-attached pop-up tent. She mentioned that the convenience of setting up the tent in minutes allowed her to spend more time enjoying nature and less time dealing with complicated tent assembly. Additionally, she appreciated the cost-saving benefits, as she no longer had to pay extra fees for her tent at campgrounds.
Summary
A car-attached pop-up tent offers the perfect solution for outdoor enthusiasts seeking convenience and cost savings. With its easy setup and attachment to your vehicle, it eliminates the hassle of traditional tents. By avoiding campground fees, reducing fuel costs, and eliminating the need for additional camping gear, you can save up to $80 on your camping adventures. Real-life examples and customer satisfaction further support the benefits of these innovative tents. So why wait? Invest in a car-attached pop-up tent today and start enjoying stress-free camping while saving money!