Improving Python Code Performance with Concurrency and Parallelism
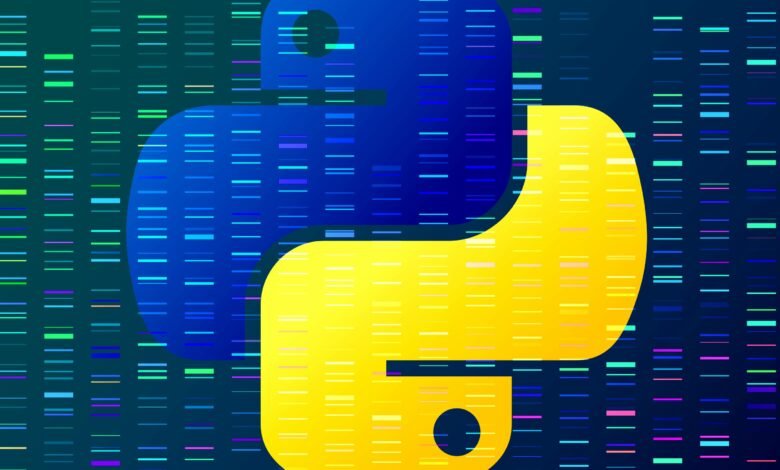
Python is a popular programming language known for its simplicity and readability. However, when it comes to performance, Python can sometimes be slower compared to other languages like C or Java. Fortunately, there are techniques and tools available to improve Python code performance, such as concurrency and parallelism. In this article, we will explore how concurrency and parallelism can be used to optimize Python code and achieve better performance.
Understanding Concurrency and Parallelism
Concurrency and parallelism are two concepts that are often used interchangeably, but they have distinct meanings in the context of programming.
Concurrency refers to the ability of a program to execute multiple tasks simultaneously. It allows different parts of a program to make progress independently, even if they are not executed at the same time. Concurrency is particularly useful when dealing with I/O-bound tasks, such as reading from or writing to a file or making network requests.
Parallelism, on the other hand, involves executing multiple tasks simultaneously by utilizing multiple processors or cores. It is especially beneficial for CPU-bound tasks, where the program can take advantage of the available hardware resources to perform computations in parallel.
Using Concurrency for I/O-Bound Tasks
Python provides several libraries and modules that enable concurrent programming. One of the most popular ones is the asyncio
module, which allows you to write asynchronous code using coroutines and event loops.
By using asyncio
, you can write code that performs I/O-bound tasks concurrently, improving the overall performance of your program. Here’s an example:
import asyncio
async def fetch_data(url):
# Simulate an I/O-bound task
await asyncio.sleep(1)
print(f"Fetched data from {url}")
async def main():
urls = ["https://example.com", "https://google.com", "https://github.com"]
tasks = [fetch_data(url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
In this example, the fetch_data
function simulates an I/O-bound task by sleeping for 1 second. By using asyncio
and await
, we can execute multiple fetch_data
calls concurrently, reducing the total execution time.
Utilizing Parallelism for CPU-Bound Tasks
Python also provides libraries and modules for parallel programming, allowing you to take advantage of multiple processors or cores. One such library is multiprocessing
, which enables you to spawn multiple processes and distribute the workload across them.
Here’s an example of using multiprocessing
to parallelize a CPU-bound task:
import multiprocessing
def calculate_square(n):
return n * n
def main():
numbers = [1, 2, 3, 4, 5]
with multiprocessing.Pool() as pool:
results = pool.map(calculate_square, numbers)
print(results)
if __name__ == "__main__":
main()
In this example, the calculate_square
function performs a CPU-bound task of calculating the square of a number. By using multiprocessing.Pool
and pool.map
, we can distribute the workload across multiple processes, effectively utilizing the available CPU cores and reducing the execution time.
Combining Concurrency and Parallelism
In some cases, it is beneficial to combine both concurrency and parallelism to achieve the best performance. For example, if you have a program that needs to fetch data from multiple URLs and perform CPU-bound computations on the fetched data, you can use both asyncio
and multiprocessing
to optimize the code.
Here’s an example that demonstrates the combination of concurrency and parallelism:
import asyncio
import multiprocessing
async def fetch_data(url):
# Simulate an I/O-bound task
await asyncio.sleep(1)
return url
def calculate_square(n):
# Simulate a CPU-bound task
return n * n
async def main():
urls = ["https://example.com", "https://google.com", "https://github.com"]
tasks = [fetch_data(url) for url in urls]
fetched_data = await asyncio.gather(*tasks)
with multiprocessing.Pool() as pool:
results = pool.map(calculate_square, fetched_data)
print(results)
asyncio.run(main())
In this example, we first use asyncio
to fetch data from multiple URLs concurrently. Once the data is fetched, we use multiprocessing
to distribute the CPU-bound computation across multiple processes. By combining both techniques, we can achieve better performance and reduce the overall execution time.
Summary
Improving Python code performance can be achieved through the use of concurrency and parallelism. Concurrency allows for the simultaneous execution of I/O-bound tasks, while parallelism enables the distribution of CPU-bound tasks across multiple processors or cores. By utilizing libraries such as asyncio
and multiprocessing
, Python developers can optimize their code and achieve better performance.
Key takeaways:
- Concurrency allows for the simultaneous execution of tasks, particularly useful for I/O-bound operations.
- Parallelism enables the distribution of tasks across multiple processors or cores, beneficial for CPU-bound operations.
- Python provides libraries like
asyncio
andmultiprocessing
to implement concurrency and parallelism. - Combining concurrency and parallelism can further enhance performance.
By leveraging these techniques, Python developers can optimize their code and achieve significant performance improvements, making Python a more viable choice for computationally intensive tasks.